Noter
Cliquez ici pour télécharger l'exemple de code complet
Styles de boîte personnalisés #
Cet exemple illustre l'implémentation d'un fichier BoxStyle
. Les ConnectionStyle
s et ArrowStyle
s personnalisés peuvent être définis de la même manière.
from matplotlib.patches import BoxStyle
from matplotlib.path import Path
import matplotlib.pyplot as plt
Les styles de boîte personnalisés peuvent être implémentés en tant que fonction qui prend des arguments spécifiant à la fois une boîte rectangulaire et la quantité de "mutation", et renvoie le chemin "muté". La signature spécifique est celle ci-
custom_box_style
dessous.
Ici, nous retournons un nouveau chemin qui ajoute une forme de "flèche" à gauche de la boîte.
Le style de boîte personnalisé peut ensuite être utilisé en passant
à .bbox=dict(boxstyle=custom_box_style, ...)
Axes.text
def custom_box_style(x0, y0, width, height, mutation_size):
"""
Given the location and size of the box, return the path of the box around
it.
Rotation is automatically taken care of.
Parameters
----------
x0, y0, width, height : float
Box location and size.
mutation_size : float
Mutation reference scale, typically the text font size.
"""
# padding
mypad = 0.3
pad = mutation_size * mypad
# width and height with padding added.
width = width + 2 * pad
height = height + 2 * pad
# boundary of the padded box
x0, y0 = x0 - pad, y0 - pad
x1, y1 = x0 + width, y0 + height
# return the new path
return Path([(x0, y0),
(x1, y0), (x1, y1), (x0, y1),
(x0-pad, (y0+y1)/2), (x0, y0),
(x0, y0)],
closed=True)
fig, ax = plt.subplots(figsize=(3, 3))
ax.text(0.5, 0.5, "Test", size=30, va="center", ha="center", rotation=30,
bbox=dict(boxstyle=custom_box_style, alpha=0.2))
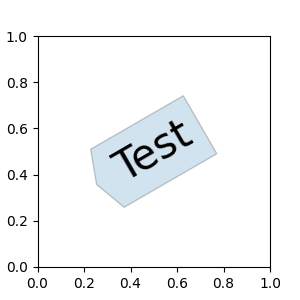
Text(0.5, 0.5, 'Test')
De même, les styles de boîte personnalisés peuvent être implémentés en tant que classes qui implémentent
__call__
.
Les classes peuvent ensuite être enregistrées dans le BoxStyle._style_list
dict, ce qui permet de spécifier le style de boîte sous forme de chaîne,
. Notez que cet enregistrement repose sur des API internes et n'est donc pas officiellement pris en charge.bbox=dict(boxstyle="registered_name,param=value,...", ...)
class MyStyle:
"""A simple box."""
def __init__(self, pad=0.3):
"""
The arguments must be floats and have default values.
Parameters
----------
pad : float
amount of padding
"""
self.pad = pad
super().__init__()
def __call__(self, x0, y0, width, height, mutation_size):
"""
Given the location and size of the box, return the path of the box
around it.
Rotation is automatically taken care of.
Parameters
----------
x0, y0, width, height : float
Box location and size.
mutation_size : float
Reference scale for the mutation, typically the text font size.
"""
# padding
pad = mutation_size * self.pad
# width and height with padding added
width = width + 2.*pad
height = height + 2.*pad
# boundary of the padded box
x0, y0 = x0 - pad, y0 - pad
x1, y1 = x0 + width, y0 + height
# return the new path
return Path([(x0, y0),
(x1, y0), (x1, y1), (x0, y1),
(x0-pad, (y0+y1)/2.), (x0, y0),
(x0, y0)],
closed=True)
BoxStyle._style_list["angled"] = MyStyle # Register the custom style.
fig, ax = plt.subplots(figsize=(3, 3))
ax.text(0.5, 0.5, "Test", size=30, va="center", ha="center", rotation=30,
bbox=dict(boxstyle="angled,pad=0.5", alpha=0.2))
del BoxStyle._style_list["angled"] # Unregister it.
plt.show()
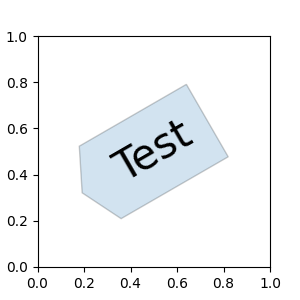